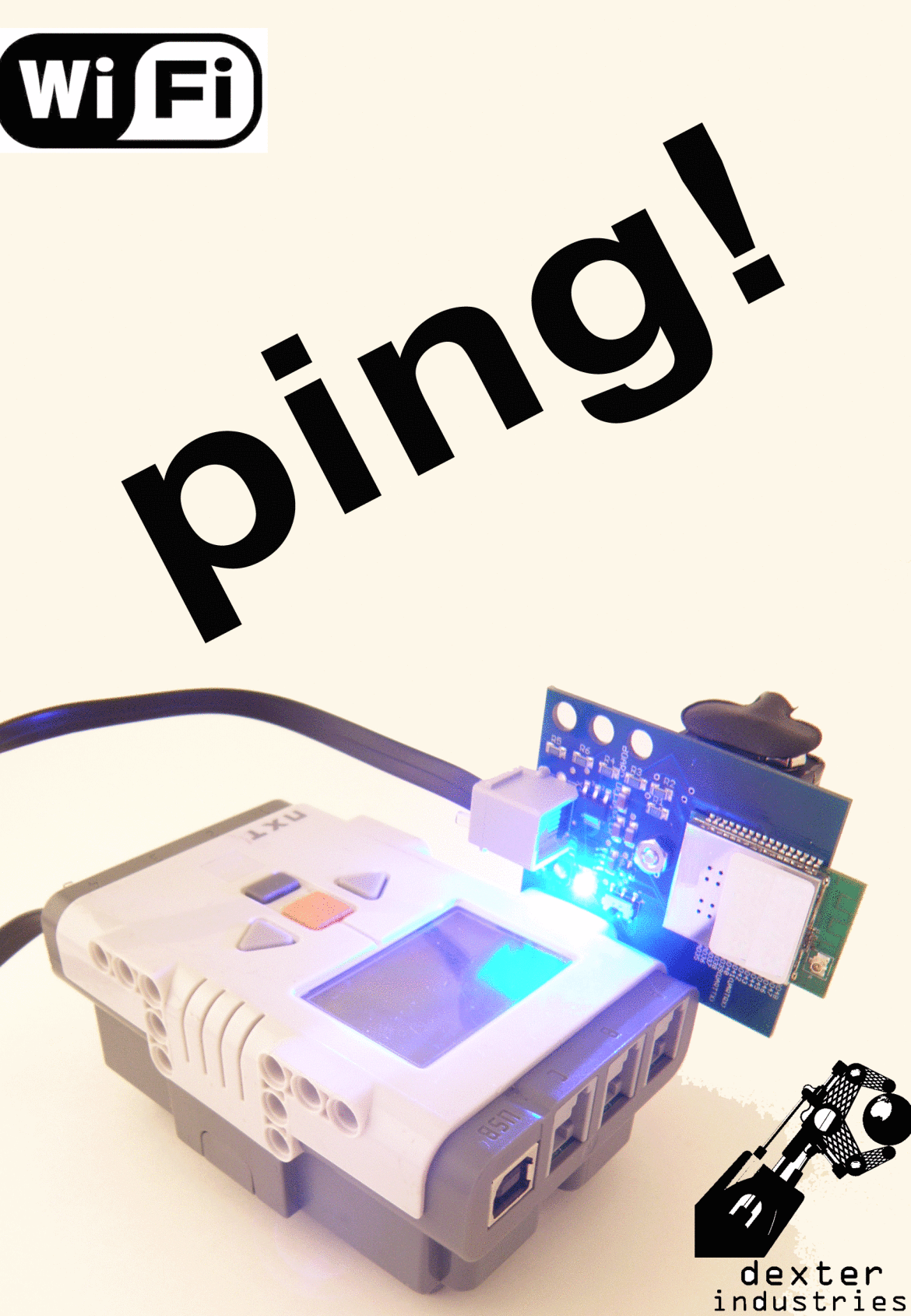
The wifi sensor comes packed with functionality. One important function for projects is to find a websites’ IP number. Another is to ping an IP number to test the speed of your connection.
In today’s installment of Wifi Week, we’re going to talk about how to get the IP number of a website, and ping it, with the Dexter Industries Wifi Sensor for LEGO MINDSTORMS.
Like the previous posts we’ve done this week, the example at the bottom of the page is written in RobotC, but the principles are universal for the Wifi Sensor.
Like some of the previous posts we’ve done before, getting an IP and pinging that IP is a very easy process.
Get an IP Address
Getting an IP address is also referred to as a DNS Lookup, or Domain Name Server Lookup. When we type in a domain, such as http://www.dexterindustries.com, the name “www.dexterindustries.com” is only for human-consumption. As soon as you type that in, the network converts the domain name into an IP address (Internet Protocol). For example, the address www.dexterindustries.com has (when we checked last) an IP number of
To get an address, we simply use the command:
AT+DNSLOOKUP=www.dexterindustries.com
The command returns the IP number of our website. In the example below, the IP number is fed into an array we use later.
Ping an IP Address
Now that we’ve got an IP address, we can use it for many things, such as posting HTTP messages on websites or even posting a twitter message (coming in two days!).
Let’s ping the dexterindustries.com website now. To do this, we use the command:
AT+PING=<IP NUMBER>
Where <IP NUMBER> is the IP of the website we’re trying to access.
Sending this command will continuously ping the IP until either the power is shut off, or you send the command
<ESC> E
Sending <ESC>E will stop the pinging.
See Examples
DIWIFI_Ping.c
[sourcecode language=”cpp”] //////////////////////////////////////////////////////////////////////////////////// Ping.c
// This program demonstrates two functions: getting the IP
// number of an http domain, and then pinging the IP number.
//
// This program is written for the Dexter Industries DIWIFI
// More information can be found at www.dexterindustries.com
// More information on the Wifi sensor at http://dexterindustries.com/manual/wifi/
//
// Modify the array "DNS_Name" to get to a specific domain.
///////////////////////////////////////////////////////////////////////////////////
// ubyte DNS_Name[] = {‘w’,’w’,’w’,’.’,’l’,’e’,’g’,’o’,’.’,’c’,’o’,’m’};
ubyte DNS_Name[] = {‘w’,’w’,’w’,’.’,’d’,’e’,’x’,’t’,’e’,’r’,’i’,’n’,’d’,’u’,’s’,’t’,’r’,’i’,’e’,’s’,’.’,’c’,’o’,’m’};
ubyte IP_num[64];
#include "drivers/common.h"
#include "DIWIFI-Ping.h"
task main(){
get_IP_address(); // Function gets the IP number.
while(true){
ping_IP(); // Repeatedly ping the IP number we got for Dexterinudstries.com
}
}
DIWIFI_Ping.h
[sourcecode language=”cpp”] /////////////////////////// DIFWIFI Ping Functions
/////////////////////////
ubyte BytesRead[8];
typedef ubyte buff_t[512];
buff_t buffer;
ubyte newline[] = {0x0D};
// writeArray function writes an array to the RawHS function
// and prints it out in the debug stream.
void writeArray(int indx)
{
string buff_out;
char buff_iter;
int index = indx;
nxtWriteRawHS(buffer, index); // Write to high speed port
writeDebugStream("%c", newline); // Give us a new line to make it look clean.
for(int t = 0; t < index; t++) // Write to DebugStream
{
buff_iter = buffer[t]; // Load up the specific iteration.
StringFromChars(buff_out, buff_iter); // Make it a string.
writeDebugStream(buff_out); // Now print to DebugStream
}
}
void debugnxtWriteRawHS(const ubyte &pData, const short nLength)
{
string tmpString;
ubyte buff[30];
memset(buff[0], 0, 30);
memcpy(buff[0], pData, nLength);
StringFromChars(tmpString, buff);
writeDebugStream("%s", tmpString);
nxtWriteRawHS(pData, nLength);
}
////////////////////////////////////////////////////////////////////////////////////////////////////////
// Receive Bytes
// Reads whatever is in the buffer and prints to debug
////////////////////////////////////////////////////////////////////////////////////////////////////////
void Receive(bool wait=false)
{
if (wait)
while (nxtGetAvailHSBytes() == 0) wait1Msec(5);
while (nxtGetAvailHSBytes() > 0) {
nxtReadRawHS(BytesRead[0], 1);
writeDebugStream("%c", BytesRead[0]);
wait1Msec(2);
}
}
int appendToBuff(buff_t &buf, const long index, const ubyte &pData, const long nLength)
{
if (index == 0) memset(buf, 0, sizeof(buf));
memcpy(buf[index], pData, nLength);
return index + nLength;
}
////////////////////////////////////////////////////////////////////////////////////////////////////////
// This function reads an IP address and puts it into a
// ubyte array.
////////////////////////////////////////////////////////////////////////////////////////////////////////
void Read_IP()
{
int t = 0;
while (nxtGetAvailHSBytes() == 0) wait1Msec(5);
while (nxtGetAvailHSBytes() > 0) {
nxtReadRawHS(BytesRead[0], 1);
if(t>4 && BytesRead != 13 && BytesRead[0] != ‘O’ && BytesRead[0] != ‘K’){
IP_num[t-5] = BytesRead[0];
}
wait1Msec(2);
t++;
}
}
//////////////////////////////////////////////////////////////////////////////////////////////////////////
// This will get the IP address of a specific domain name.
//////////////////////////////////////////////////////////////////////////////////////////////////////////
void get_IP_address() {
int index = 0;
ubyte DNSlookupCmd[] = {‘A’,’T’,’+’,’D’,’N’,’S’,’L’,’O’,’O’,’K’,’U’,’P’,’=’};
index = appendToBuff(buffer, index, DNSlookupCmd, sizeof(DNSlookupCmd));
index = appendToBuff(buffer, index, DNS_Name, sizeof(DNS_Name));
index = appendToBuff(buffer, index, newline, sizeof(newline));
writeArray(index);
Read_IP();
}
//////////////////////////////////////////////////////////////////////////////////////////////////////////
// Function pings an IP
//////////////////////////////////////////////////////////////////////////////////////////////////////////
void ping_IP() {
Receive();
int index = 0;
ubyte listen_cmd[] = {‘a’,’t’,’+’,’p’,’i’,’n’,’g’,’=’};
index = appendToBuff(buffer, index, listen_cmd, sizeof(listen_cmd));
index = appendToBuff(buffer, index, IP_num, 14);
index = appendToBuff(buffer, index, newline, sizeof(newline));
writeArray(index);
while(true){
Receive(true);
}
}
3 Comments
-
Hi,
Which version of RobotC is used for the WIFI programs. I am using the latest version and getting all kinds of errors. They worked some time back??
Best wishes J
-
Hey Jakob,
These examples use a much older version, 2.x I think. They are now way out of date.
Best,
John
-
Leave a reply
You must be logged in to post a comment.